- 1 Why Developers Worldwide Choose Python?
-
2
Top 10 Python Interview Questions
- 2.1 1. Explain Python’s Key Features
- 2.2 2. What Are Python’s Data Types?
- 2.3 3. Explain Python’s Memory Management
- 2.4 4. What Are Python Decorators?
- 2.5 5. How Does Python Handle Multithreading?
- 2.6 6. What Are Python List Comprehensions?
- 2.7 7. What are Python’s Modules and Packages?
- 2.8 8. How to Handle Exceptions in Python?
- 2.9 9. What Are Lambda Functions in Python?
- 2.10 10. What is the Difference Between Deep Copy and Shallow Copy in Python?
- 3 Practical Tips to Ace Python Interviews
- 4 Wrapping It Up
Python continues to dominate the programming landscape as one of the most widely embraced and versatile languages. Its straightforward syntax and adaptability have made it a go-to option across industries, particularly in fields like machine learning, web development, and process automation. By 2025, the demand for Python developers is soaring, driven by businesses seeking cutting-edge yet scalable solutions.
For job seekers, Python interviews are becoming increasingly demanding, focusing not only on code clarity but also on problem-solving techniques, optimization strategies, and mastery of Python’s unique features. Many interview questions are inspired by real-world scenarios, such as managing concurrent processes or creating efficient data handling systems. Preparing for these challenges is about more than landing a job; it’s an opportunity to showcase how Python’s flexibility can drive innovation and solve complex problems.
In this article, we’ll explore the top 10 Python interview questions for 2025. These questions reflect the latest trends and challenges in the tech industry, offering clear explanations and practical examples to help you feel ready for your next interview.
Why Developers Worldwide Choose Python?
Python has earned a special place in the programming world, and its appeal only continues to grow. Its simplicity, combined with unmatched versatility, sets it apart from other languages. By 2024, Python’s popularity reached new heights, even overtaking JavaScript on GitHub as developers embraced its use in data science, machine learning, and other transformative fields.
The numbers tell an impressive story, but the love for Python isn’t just statistical. The 2024 Stack Overflow Developer Survey ranked Python as one of the most admired and sought-after languages, underscoring its impact on programmers everywhere.
Python’s influence goes far beyond borders. A 2023 survey by the Python Software Foundation and JetBrains gathered responses from over 25,000 developers spanning nearly 200 countries. This highlighted not only the language’s widespread adoption but also the strength of its global community a key factor behind its sustained success.
What makes Python truly exceptional is its balance of simplicity and power. Its clean, human-readable syntax makes it perfect for beginners starting their coding journey. Meanwhile, seasoned professionals leverage its extensive libraries and frameworks to tackle advanced challenges like building sophisticated web apps or automating complex workflows. This dual nature accessible to novices yet robust for experts cements Python as a favorite for developers of all levels.
Top 10 Python Interview Questions
1. Explain Python’s Key Features
Python is a widely-used, high-level programming language known for its simplicity and versatility. It is favored by developers for its broad applicability across domains like web development, machine learning, data science, and automation. Python’s popularity is largely due to its rich set of features that enhance development speed and flexibility. Below are its key features explained:
- Ease of Learning: Python’s straightforward syntax and minimal keywords make it beginner-friendly, allowing developers to focus on solving problems rather than deciphering the language.
- Open Source: Python is free to use, modify, and distribute, fostering a large community that contributes to its growth.
- Cross-Platform: Python applications run seamlessly on Linux, macOS, and Windows without modification, making it an excellent choice for diverse environments.
- Interpreted Language: Python processes code line-by-line, which simplifies testing and debugging, enabling developers to iterate quickly.
- Object-Oriented and Multi-Paradigm: Python supports object-oriented, procedural, and functional programming styles, offering flexibility to address a wide range of problems.
- Standard Library: It provides built-in modules for common tasks like web development, database management, and file handling.
- Extensibility: Python can integrate with other languages and tools, making it suitable for specialized tasks.
These features position Python as a powerful tool for both rapid prototyping and complex application development.
2. What Are Python’s Data Types?
Python provides a wide range of built-in data types, making it a versatile programming language suitable for different use cases. Each data type is designed to handle specific types of information efficiently. Below is a comprehensive overview of Python’s core data types:
- Integers: Represent whole numbers, such as 10 or -5. They are often used in mathematical operations like counting or indexing.
- Floats: Represent decimal numbers, such as 3.14 or -0.01. Floats are commonly used in financial calculations or scientific computations.
- Strings: Used to store textual data. Strings are enclosed in single, double, or triple quotes, such as “Hello” or ‘Python’. They support numerous methods like .upper() or .split() for text manipulation.
- Lists: Ordered and mutable collections of items. They can hold heterogeneous elements like [1, “two”, 3.0]. Lists are ideal for storing sequences that require frequent modification.
- Tuples: Ordered but immutable collections. A tuple, such as (1, 2, 3), is useful when data integrity is critical.
- Sets: Unordered collections of unique items, such as {1, 2, 3}. Sets are commonly used for removing duplicates or performing set operations like union and intersection.
- Dictionaries: Represent key-value pairs. For example, {“name”: “Alice”, “age”: 25} is a dictionary. They are invaluable for mapping relationships or storing configurations.
- Booleans: Represent True or False values, often used in conditional statements.
- NoneType: Represents the absence of a value or a null value, denoted by None.
These data types are the foundation of Python programming, enabling developers to model and manipulate data effectively. Choosing the right data type based on the context ensures efficiency and clarity in code design, making Python a preferred language for diverse applications.
3. Explain Python’s Memory Management
Python’s memory management system is a combination of automatic processes that efficiently allocate, track, and reclaim memory to ensure smooth program execution. Below is a detailed explanation:
Memory Allocation:
Python uses two primary memory areas:
- Stack Memory: Handles function calls and local variables.
- Heap Memory: Stores objects and data structures.
Memory allocation for objects occurs dynamically, meaning Python assigns memory during runtime. Developers don’t need to specify or manage memory allocation manually.
Garbage Collection:
Python has a built-in garbage collector to reclaim unused memory. When objects are no longer referenced, the garbage collector removes them to free up space. This process is automatic but can also be triggered manually using the gc module:
import gc
gc.collect() # Manually trigger garbage collection
Reference Counting:
Every object in Python has a reference count, which tracks how many references point to the object. When the reference count drops to zero, the object becomes eligible for garbage collection.
Handling Cyclic References:
Cyclic references occur when two objects reference each other but are otherwise unused. The garbage collector identifies and breaks such cycles.
Example:
import gc
class Node:
def __init__(self, name):
self.name = name
self.ref = None
a = Node('A')
b = Node('B')
a.ref = b
b.ref = a # Cyclic reference
del a
del b # Objects still exist due to cyclic reference
gc.collect() # Removes cycles and reclaims memory
Python’s memory management system simplifies development by automating these processes, making the language developer-friendly while ensuring optimal performance.
4. What Are Python Decorators?
Decorators in Python are a powerful feature that allows you to modify or extend the behavior of functions or methods without altering their actual code. They enable the application of reusable functionality across multiple functions, such as timing, caching, logging, and authentication.
Understanding Python Decorators
A decorator is essentially a function that takes another function as an argument and returns a new function that typically extends or alters the behavior of the original function. This is achieved by wrapping the original function within another function, known as a wrapper.
Basic Syntax
The syntax for defining a decorator involves using the @decorator_name notation above the function definition you wish to decorate. Here’s a simple example:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
In this example, when say_hello() is called, it will first execute the code in wrapper(), demonstrating how decorators can add behavior before and after the original function call.
Use Cases for Decorators
Decorators are commonly used for various purposes, including:
- Logging: Tracking function calls and their parameters.
- Authentication: Checking user permissions before executing a function.
- Caching: Storing results of expensive function calls to improve performance.
- Timing: Measuring how long a function takes to execute.
Each of these use cases enhances code readability and modularity by separating concerns without modifying existing code directly.
Types of Decorators
- Function Decorators: These are the most common type and apply to individual functions.
- Class Decorators: These extend or modify class behavior instead of functions.
- Decorators with Arguments: These allow passing parameters to customize their behavior further.
Example of a Function Decorator with Arguments
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
In this example, the greet function will be called three times due to the repeat decorator.
5. How Does Python Handle Multithreading?
Python handles multithreading primarily through the threading module, which provides a simple and intuitive API for creating and managing threads. Here’s how it works:
Thread Creation: To create a new thread, you instantiate an object of the Thread class, specifying the target function to execute and any necessary arguments.
For example:
import threading
def task():
print("Task is running")
thread = threading.Thread(target=task)
Starting Threads: Once a thread is created, it can be started using the start() method. This method initiates the thread’s activity, allowing it to run concurrently with other threads.
thread.start()
Thread Execution: Threads run in parallel within the same process, sharing the same memory space. This allows for efficient communication between threads but can lead to issues like race conditions if not managed properly.
Global Interpreter Lock (GIL): Despite enabling multithreading, Python’s GIL prevents true parallel execution of threads in CPU-bound tasks. This means that while threads can run concurrently, only one thread executes Python bytecode at a time. Therefore, multithreading in Python is most effective for I/O-bound operations rather than CPU-bound tasks.
Thread Management: The threading module also provides methods for managing threads, such as join(), which blocks the calling thread until the thread whose join() method is called is terminated.
Overall, Python’s multithreading capabilities allow for improved program responsiveness and efficiency, especially in scenarios involving I/O operations. However, developers must be cautious of potential concurrency issues due to the GIL and shared data access.
6. What Are Python List Comprehensions?
Python list comprehensions provide a concise way to create lists by applying an expression to each item in an iterable, such as a list or a range. This feature allows for cleaner and more readable code compared to traditional looping methods.
Syntax of List Comprehensions
The basic syntax for a list comprehension is:
new_list = [expression for item in iterable if condition]
- expression: This defines what to include in the new list.
- item: Each element from the iterable.
- iterable: Any iterable object (like a list, tuple, or string).
- condition: An optional filter that determines whether the item should be included in the new list.
Examples
- Creating a New List:
fruits = ["apple", "banana", "cherry", "kiwi", "mango"]
newlist = [x for x in fruits if "a" in x]
print(newlist)
# Output: ['apple', 'banana', 'mango']
- Using Expressions:
numbers = [1, 2, 3, 4, 5]
squares = [x**2 for x in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
- With Conditions:
even_numbers = [x for x in range(10) if x % 2 == 0]
print(even_numbers) # Output: [0, 2, 4, 6, 8]
List comprehensions not only simplify the syntax but also enhance performance by reducing the overhead of function calls associated with traditional loops. They are particularly useful for generating new lists based on existing data while maintaining clarity and conciseness in code.
7. What are Python’s Modules and Packages?
Python’s modules and packages are essential for organizing and structuring code, allowing developers to manage complexity in their applications effectively.
Modules
A module in Python is a single file containing Python code, which can include functions, classes, and variables. Modules help break down large programs into smaller, manageable sections. They can be imported into other Python scripts or modules using the import statement.
Example of a Module
For instance, consider a file named math_utils.py:
# math_utils.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
You can import this module in another script:
import math_utils
result = math_utils.add(5, 3)
print(result) # Output: 8
Packages
A package is a collection of related modules organized in a directory hierarchy. It must contain an __init__.py file (which can be empty) to indicate to Python that the directory should be treated as a package. Packages allow for better organization of code and enable grouping of related functionalities under a common namespace.
Example of a Package
Consider a package named my_package that contains two modules: module1.py and module2.py.
my_package/
__init__.py
module1.py
module2.py
You can import modules from this package as follows:
from my_package import module1
result = module1.some_function()
Key Differences
Aspect | Module | Package |
Definition | A single file with Python code | A directory containing multiple modules |
Structure | Contains functions, classes, variables | Organized with an __init__.py file |
Purpose | Code organization | Code distribution and reuse |
Examples | math, random, datetime | numpy, pandas, matplotlib |
In summary, modules and packages are fundamental concepts in Python that facilitate code organization, promote reusability, and enhance maintainability in software development.
8. How to Handle Exceptions in Python?
In Python, exceptions are errors that occur during the execution of a program, disrupting its normal flow. To handle these exceptions gracefully, Python provides a structured approach using try and except blocks.
Handling Exceptions
Basic Syntax
The basic structure for handling exceptions involves placing potentially error-generating code inside a try block, followed by one or more except blocks to catch and handle specific exceptions.
try:
# Code that may cause an exception
result = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
In this example, attempting to divide by zero raises a ZeroDivisionError, which is caught and handled in the corresponding except block.
Catching Specific Exceptions
You can have multiple except blocks to handle different types of exceptions separately. This allows for more precise error handling.
try:
my_list = [1, 2, 3]
print(my_list[5]) # IndexError
except IndexError:
print("Error: Index out of range.")
except ZeroDivisionError:
print("Error: Division by zero.")
Here, accessing an out-of-bounds index raises an IndexError, which is caught and handled appropriately.
Raising Exceptions
You can also raise exceptions intentionally using the raise keyword. This is useful for enforcing certain conditions in your code.
def check_positive(number):
if number < 0:
raise ValueError("Negative value provided.")
try:
check_positive(-5)
except ValueError as e:
print(e) # Output: Negative value provided.
In this example, a ValueError is raised if a negative number is passed to the function.
Using Finally
You can include a finally block that will execute regardless of whether an exception was raised or not. This is often used for cleanup actions..
try:
file = open('example.txt', 'r')
# Perform file operations
except FileNotFoundError:
print("File not found.")
finally:
file.close() # Ensures the file is closed whether an error occurred or not.
9. What Are Lambda Functions in Python?
Lambda functions in Python are small, anonymous functions defined using the lambda keyword. They can take any number of arguments but are limited to a single expression, which is evaluated and returned automatically.
Key Features of Lambda Functions
- Anonymous: Lambda functions do not have a name, making them suitable for short-term use where defining a full function is unnecessary.
- Single Expression: Unlike regular functions that can contain multiple statements, a lambda function is restricted to a single expression, which makes it concise.
- Syntax: The syntax for a lambda function is as follows:
lambda arguments: expression
Examples of Lambda Functions
- Basic Example:
add_ten = lambda x: x + 10
print(add_ten(5))
# Output: 15
- Multiple Arguments:
multiply = lambda a, b: a * b
print(multiply(3, 4))
# Output: 12
- Using with Built-in Functions:
Lambda functions are often used with functions like map(), filter(), and reduce(). For example:
numbers = [1, 2, 3, 4]
squares = list(map(lambda x: x**2, numbers))
print(squares) # Output: [1, 4, 9, 16]
When to Use Lambda Functions?
Lambda functions are particularly useful when you need a simple function for a short duration, such as when passing a function as an argument to higher-order functions (like map() or filter()), or when defining small callback functions.
In summary, lambda functions provide a streamlined way to create small, one-time-use functions in Python, enhancing code readability and efficiency in scenarios requiring simple operations.
10. What is the Difference Between Deep Copy and Shallow Copy in Python?
The difference between deep copy and shallow copy in Python primarily revolves around how objects are duplicated and how changes to those objects affect each other.
Shallow Copy
A shallow copy creates a new object but does not create copies of nested objects within the original. Instead, it populates the new object with references to the same nested objects. This means that changes made to mutable nested objects in the shallow copy will reflect in the original object, as both share references to the same nested objects.
Example:
import copy
original = [[1, 2, 3], [4, 5, 6]]
shallow_copied = copy.copy(original)
shallow_copied[0][0] = 'Changed'
print(original) # Output: [['Changed', 2, 3], [4, 5, 6]]
print(shallow_copied) # Output: [['Changed', 2, 3], [4, 5, 6]]
Deep Copy
A deep copy, on the other hand, creates a new object and recursively copies all nested objects found in the original. This results in a fully independent clone of the original object and its children. Changes made to any part of the deep copy do not affect the original object.
Example:
import copy
original = [[1, 2, 3], [4, 5, 6]]
deep_copied = copy.deepcopy(original)
deep_copied[0][0] = 'Changed'
print(original) # Output: [[1, 2, 3], [4, 5, 6]]
print(deep_copied) # Output: [['Changed', 2, 3], [4, 5, 6]]
Key Differences
Aspect | Shallow Copy | Deep Copy |
Object Duplication | Copies only the outer object; references nested objects | Recursively copies all objects; creates independent copies |
Effect of Changes | Changes in nested objects reflect in both copies | Changes in deep copied objects do not affect originals |
Performance | Generally faster due to less overhead | Slower due to recursive copying |
Understanding these differences is crucial for effective data manipulation and maintaining data integrity when working with complex data structures in Python.
Practical Tips to Ace Python Interviews
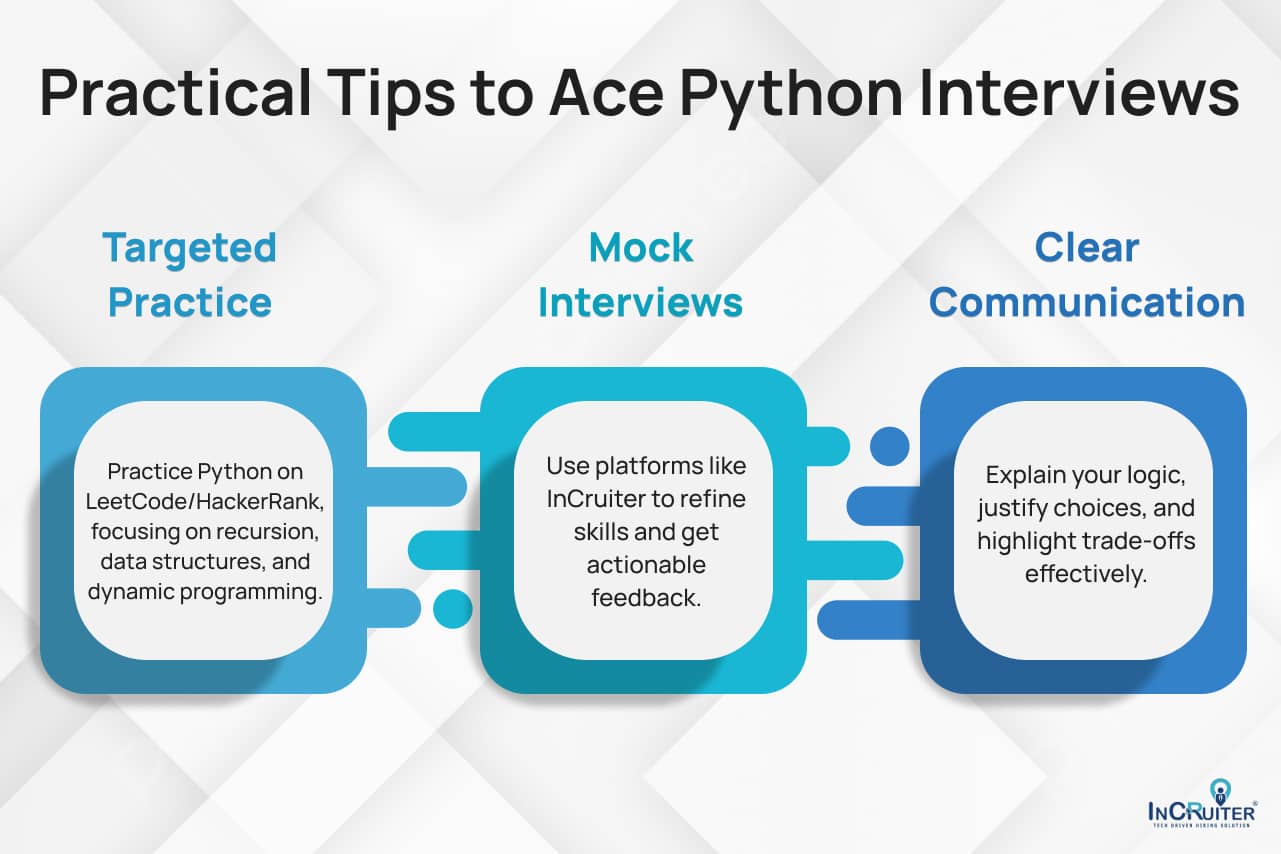
Getting ready for Python interviews means more than just knowing the basics or solving coding tasks. Here are some hands-on, advanced tips to boost your preparation and stand out during interviews:
Practice with Purpose on Problem-Solving Platforms
Use platforms such as LeetCode, HackerRank, or InterviewBit to sharpen your skills. Instead of picking problems randomly, search for challenges linked to specific companies or Python-related roles. Focus on topics like recursion, data structures, and dynamic programming since these are frequent in interviews. To build speed and confidence, time your solutions and read explanations for areas where your approach could improve.
Experience Real Interviews Mock Sessions
Excelling in interviews isn’t just about writing code; it’s about solving problems under pressure. InCruiter offers mock interview setups that replicate the actual interview experience. These sessions help you understand how panels assess your coding skills, logic, and overall approach. Detailed feedback highlights areas to improve, like debugging, optimizing your solutions, or handling Python-specific quirks such as managing edge cases or using libraries effectively.
Explain Your Thinking Clearly
In interviews, how you solve a problem is as important as solving it. Walk the interviewer through your thought process. Start by confirming the problem’s requirements and limitations. For example, explain why you’d use a dictionary for fast lookups or opt for a generator to save memory. If you optimize your solution, discuss the trade-offs between speed and simplicity so the panel understands your choices.
Wrapping It Up
Getting ready for Python interviews is about more than just knowing the syntax. It’s about combining technical know-how, problem-solving abilities, and the confidence to explain your ideas clearly. By diving into Python’s essential concepts and practicing coding tasks that mirror real-life scenarios, you’ll make yourself stand out as a capable and prepared candidate.
Take time to understand the reasoning behind every solution you come across. Interviewers often focus on your thought process rather than just the final answer. Regular mock interviews and steady practice can boost your confidence and help reduce common mistakes.
If you’re looking for more helpful advice, check out the InCruiter blog. It’s packed with resources to guide you on your journey to acing interviews and securing your dream role.